Description
Given a 0-indexed n x n
integer matrix grid
, return the number of pairs (ri, cj)
such that row ri
and column cj
are equal.
A row and column pair is considered equal if they contain the same elements in the same order (i.e., an equal array).
Example 1:
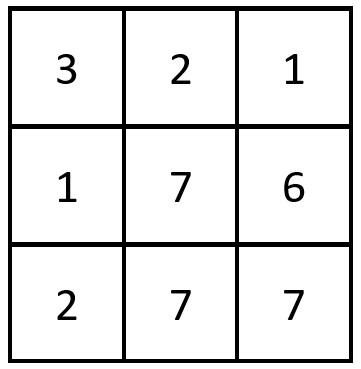
1
2
3
4
| Input: grid = [[3,2,1],[1,7,6],[2,7,7]]
Output: 1
Explanation: There is 1 equal row and column pair:
- (Row 2, Column 1): [2,7,7]
|
Example 2:
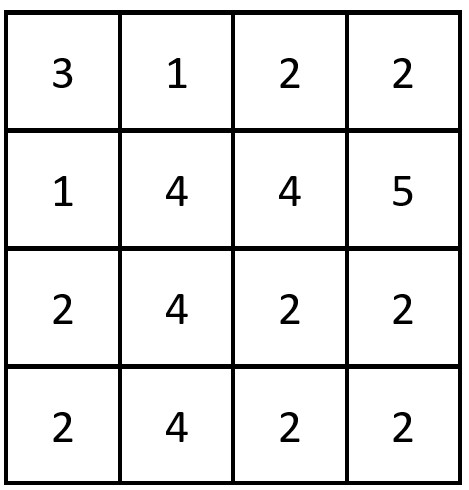
1
2
3
4
5
6
| Input: grid = [[3,1,2,2],[1,4,4,5],[2,4,2,2],[2,4,2,2]]
Output: 3
Explanation: There are 3 equal row and column pairs:
- (Row 0, Column 0): [3,1,2,2]
- (Row 2, Column 2): [2,4,2,2]
- (Row 3, Column 2): [2,4,2,2]
|
Constraints:
n == grid.length == grid[i].length
1 <= n <= 200
1 <= grid[i][j] <= 105
Solutions
Solution 1
Violent solution: Traverse each row and compare the current row with each column. If they are equal, then the two are equal.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
| class Solution {
public:
int equalPairs(vector<vector<int>>& grid) {
int res = 0, n = grid.size();
for (int row = 0; row < n; row++) {
for (int col = 0; col < n; col++) {
if (equal(row, col, grid)) {
res++;
}
}
}
return res;
}
bool equal(int row, int col, vector<vector<int>>& grid) {
int n = grid.size();
for (int i = 0; i < n; i++) {
if (grid[row][i] != grid[i][col]) {
return false;
}
}
return true;
}
};
|
Solution2
First, the rows of the matrix are put into the hash table to count the number of times. The key of the hash table can be the string after the row is concatenated, or it can use the built-in data structure of each language, and then count how many rows are equal to each column, just add it up.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| class Solution {
public:
int equalPairs(vector<vector<int>>& grid) {
int n = grid.size();
map<vector<int>, int> count;
// 将每行数据放入map
for (const auto& row : grid) {
count[row]++;
}
int res = 0;
for (int j = 0; j < n; j++) {
vector<int> arr;
for (int i = 0; i < n; i++) {
arr.emplace_back(grid[i][j]);
}
if (count.find(arr) != count.end()) {
res += count[arr];
}
}
return res;
}
};
|